Compile C Program In Dos World
How to compile and run C program in CodeBlocks. Once you created your first C program it’s time to compile and run the program. To compile and run a C program, click Build → Build and run to compile and build your C program, alternatively use the shortcut key F9. In case, your program contains any errors. This line invokes the C compiler called gcc, asks it to compile samp.c and asks it to place the executable file it creates under the name samp. To run the program, type samp (or, on some UNIX machines,./samp). On a DOS or Windows machine using DJGPP, at an MS-DOS prompt type gcc samp.c -o samp.exe.
I'm about to start learning C (I need to program something in C, to convert it into MIPS later).
I'm on Windows 8, and downloaded MinGW and set the path variable to its directory, so that the gcc or g++ commands should work in command prompt.
I copied the following Hello World example into a .c text file.
However, although I'm in the correct directory as the file, on command prompt when I type 'gcc helloWorld.c' it pauses for a couple of seconds, and then doesn't do anything. It just acts as if the program has finished and allows me to type something else in.
This is likely to be a simple mistake - I don't really understand what I'm doing. But can anyone help me out?

4 Answers
When you issue the gcc command you are actually COMPILING the source code, not RUNNING the program.The Compilation process creates an executable file to that you can run.
In the example by previous poster:
gcc -o helloWorld helloWorld.c
The -o parameter is the [o]utput name of the executable file to be created, let say 'helloworld.exe' (as you are using Windows).This should create a new file helloworld.exe, Now you can RUN that file.
You should read a little bit more on general C programming and compiling.
You can write comments with //
or /* ... */
Also to compile a c program with gcc use this:
So this should work:
Rizier123Rizier123You can either use:
which creates an executable file called executablename or use:
which will make an executable file with the default name (a.out for UNIX, I'm not sure what it is for Windows). If you look into the directory, you should be able to see this executable.
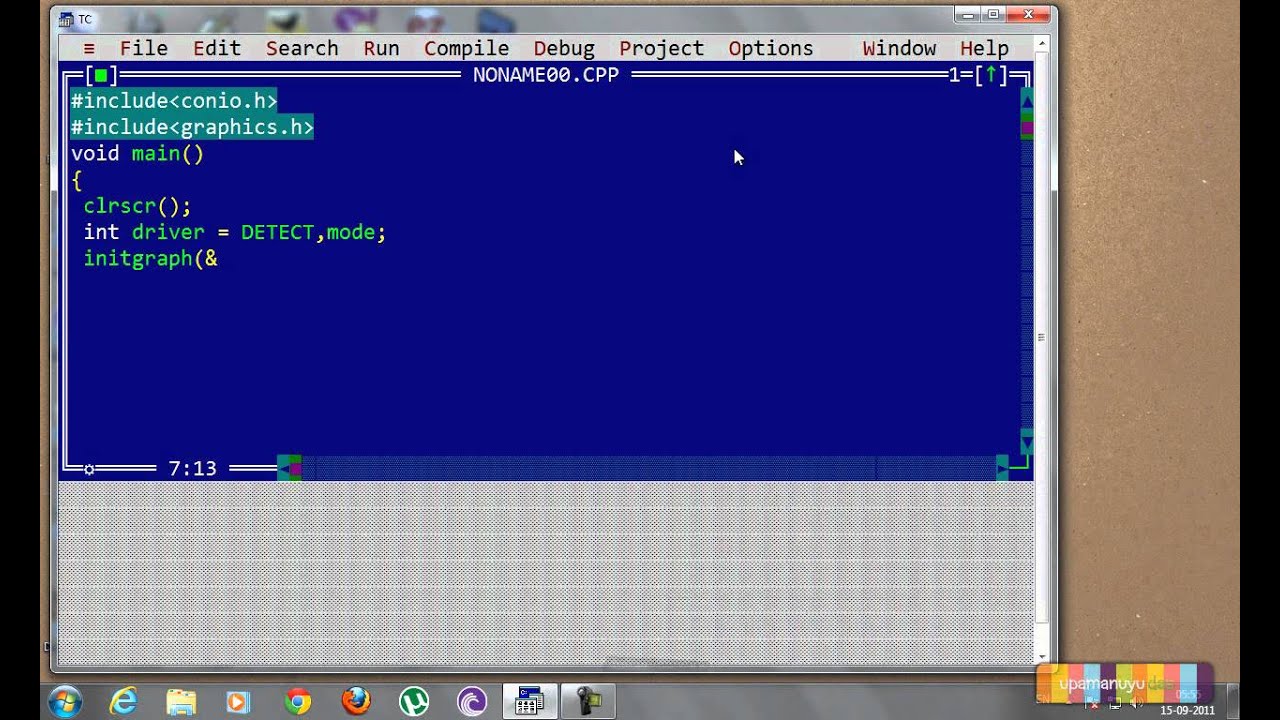
Not the answer you're looking for? Browse other questions tagged cgccmingwcommand-prompt or ask your own question.
Question
Can someone please tell me how to compile from the command line in a clear, concise way. Preferably, my programs will be written in a real text editor (such as leaf pad).
Background
I am a complete n00b when it comes to Linux, C, and the Raspberry Pi. So, the three have come together in a perfect storm to attack!
I have created a C file called main.c
in a folder. The directory is:
The C
code is a simple Hello World program - I don't need to explain it here. So, I have naturally decided to compile my C code, and it is here that I come to a loss. I type in
as told in this tutorial, but I come to an error:
When I did the nano
stuff, I came to a weird window, but I didn't know how to save what I wrote.

Thanks so much - this has been bugging me hugely.
xxmbabanexxxxmbabanexx3 Answers
Do NOT use nano
(or another text editor to put your code into) with root/sudo permissions (ie. do not edit with sudo nano
, only use nano
) if all you are doing is personal stuff that does not need superuser permissions.
To compile from the command line (assuming yourcode.c
is the name of your C file, and program
the name of the resulting program after compilation):
Write your code in your favorite editor:
- In the terminal, type
nano yourcode.c
(assuming you want to use nano); - Or use your Leafpad editor (and make sure to know where your file is saved).
- In the terminal, type
Back to the terminal, navigate to where your C file is stored.(Reminder:
ls
to list directory contents,cd
to change directory.)Compile your code with
gcc -o program yourcode.c
.Execute it with
./program
. Done!
Bonus method
If you intend on compiling/executing your program quite a lot, you may save yourself time by writing a Makefile
. Create this file with Leafpad (or, in terminal, nano Makefile
), then write:
(Make sure you presently use Tab for indents, and not spaces.) Then every time you just type make
in the terminal (or make all
, but let’s keep things short!), your program will compile and execute.
Testing
Want to make sure your GCC installation works? Copy-paste the following to your terminal:
If it echoes “Hello World”, then you’re good to go.
Compiling C programs on the raspberry pi is rather simple. First, create your program in a text editor and save it as <insert name>.c
It should be saved on the Desktop.
Next, open terminal. In it type:
This changes the directory that the terminal is looking at to Desktop. This is also where our program is stored.
This is where the interesting stuff occurs. GCC
is the compiler - it makes your code executable. -Wall
activates compiler warnings - this is extremely helpful for debugging. The next line <myName>.c
tells the computer where the code is stored. -o
is an option - it tell GCC
to compile. Lastly, <compiled name>
is the name of your new program.
Your 'Not found' error is caused by not having a ./
in front. So:
Compile C Program Linux
gcc -o ./hello ./main.c